Integrating TweetSharp-Unofficial Into Your .NET Website for Twitter Login
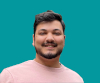
Jacob Naryan - Full-Stack Developer
Posted: Sat Aug 05 2023
Last updated: Wed Nov 22 2023
The following steps will guide you on how to integrate TweetSharp-Unofficial into your .NET website so you can integrate Twitter login.
1. Import TweetSharp
Firstly, make sure the TweetSharp library is imported into your project. This can be done by adding the following line at the top of your code:
using TweetSharp;
2. Retrieve an OAuth Request Token
The first step in the OAuth process is to retrieve a request token. This token will be used to authenticate with Twitter in the next step. The following code retrieves an OAuth request token:
public ActionResult Authorize()
{
// Step 1 - Retrieve an OAuth Request Token
TwitterService service = new TwitterService("consumerKey", "consumerSecret");
// This is the registered callback URL
OAuthRequestToken requestToken = service.GetRequestToken("http://localhost:9090/AuthorizeCallback");
// Step 2 - Redirect to the OAuth Authorization URL
Uri uri = service.GetAuthorizationUri(requestToken);
return new RedirectResult(uri.ToString(), false /*permanent*/);
}
3. Redirect to the OAuth Authorization URL
To authenticate with Twitter, you need to redirect the user to an authorization URL. The user will log in to their Twitter account and authorize your application. Then, they will be redirected back to your callback URL.
The following code redirects the user to the authorization URL:
// This URL is registered as the application's callback at http://dev.twitter.com
public ActionResult AuthorizeCallback(string oauth_token, string oauth_verifier)
{
var requestToken = new OAuthRequestToken {Token = oauth_token};
// Step 3 - Exchange the Request Token for an Access Token
TwitterService service = new TwitterService(_consumerKey, _consumerSecret);
OAuthAccessToken accessToken = service.GetAccessToken(requestToken, oauth_verifier);
// Step 4 - User authenticates using the Access Token
service.AuthenticateWith(accessToken.Token, accessToken.TokenSecret);
TwitterUser user = service.VerifyCredentials();
ViewModel.Message = string.Format("Your username is {0}", user.ScreenName);
return View();
}
4. Exchange the Request Token for an Access Token
After redirecting back to your callback URL, you will receive an OAuth token and verifier. You can exchange these for an access token, which allows you to authenticate with Twitter on behalf of the user.
The following code exchanges the request token for an access token:
OAuthAccessToken accessToken = service.GetAccessToken(requestToken, oauth_verifier);
5. Authenticate With Twitter
Finally, you authenticate with Twitter using the access token and get user details.
service.AuthenticateWith(accessToken.Token, accessToken.TokenSecret);
TwitterUser user = service.VerifyCredentials();
ViewModel.Message = string.Format("Your username is {0}", user.ScreenName);
In this step, we are also getting the authenticated user’s screen name and displaying it on our view.
That’s it! You’ve successfully set up a basic Twitter login system using TweetSharp-Unofficial in your .NET website!
Thank you for reading. If you liked this blog, check out more of my tutorials about software engineering on my personal site here.