How to Use Angular Services and Dependency Injection for Efficient Data Management
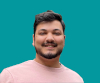
Jacob Naryan - Full-Stack Developer
Posted: Sat Jul 22 2023
Last updated: Wed Nov 22 2023
Angular Services and Dependency Injection are powerful tools that allow developers to manage data efficiently. This blog post will explain how to use these tools to create efficient data management solutions.
What are Angular Services and Dependency Injection?
Angular Services are reusable pieces of code that contain business logic or data. They can be injected into any component or directive in an Angular application in order to access their functionality. Dependency Injection (DI) is a way of managing the dependencies between different parts of an application. It allows developers to inject services into components and directives without having to manually manage the dependencies themselves.
Also, check out more Angular dev tools that you could try out.
Step 1: Create a Service
The first step is to create a service that contains the data you want to manage. This service should include methods for retrieving, updating, and deleting the data. It should also include any other business logic related to the data. For example here is a simple service with two methods to retrieve data. The first call an endpoint to get data, and the second returns the data fetched earlier by the first method.
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable, Subject } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class HttpService {
constructor(private http: HttpClient) { }
private data: any;
private _data: new Subject<any>();
$data = this._data.asObservable();
getData(): Observable<any> {
return this.http.get('http://my-url-here.com/data').subscribe({
next: (response) => {
this.data = response;
this._data.next(response)
},
error: (response) => {
console.log(response)
}
});
} //end getData
retreiveData(): any{
return this.data;
}
}
💡 Note: If you need to reuse the same logic across multiple projects, you could use a tool like Bit to encapsulate common logic and share it as packages across all your projects, reducing code duplication and cutting down on boilerplate. Bit also comes with an integrated dev environment that lets you test, document, version, and track changes to your components over time, making it easier to maintain your components, manage dependencies and ensure compatibility across your applications.
Learn more:
Step 2: Register the Service
Once the service is created, it needs to be registered with the Angular dependency injector. This can be done in the app.module.ts file, or any other module file that will be using the service.
@NgModule({
...
providers: [
...
HttpService
],
...
})
export class AppModule {}
Step 3: Inject the Service
The next step is to inject the service into any component or directive where it will be used. This can be done by adding an argument to the constructor method of the component or directive with the same type as the service. The dependency injector will then automatically inject the service into the component or directive when it is created.
import { Component } from '@angular/core';
import { HttpService } from './http.service'; // import the service here
@Component({ ... })
export class MyComponent {
constructor(private httpService: HttpService) { } // inject the service here
...
}
Step 4: Use the Service
Once the service has been injected into the component or directive, it can be used to access and manage data efficiently. Here we are setting up local variables in the component for the app data, and subscribing to the result of the API call we set up in our service. Then in the ngOnInit function, we are calling the retrieve data function to first check if our data has already been fetched, if not, we will trigger the API call. Now you can use the data variable in your components template file to show dynamic data on your screen.
import { Component } from '@angular/core';
import { HttpService } from './http.service'; // import the service here
@Component({ ... })
export class MyComponent {
data: any
$data: Subscription
constructor(private httpService: HttpService) {
this.$data = this.httpService.$data.subscribe((response) => {this.data = response});
}
ngOnInit(){
var data = this.httpervice.retreiveData();
if(data == null){
this.httpService.getData();
}
}
}
Conclusion
Using Angular Services and Dependency Injection is an effective way of managing data efficiently in an Angular application. By following these steps, developers can easily create services, register them with the dependency injector, and inject them into components and directives in order to access their functionality.
Thank you for reading. If you liked this blog check out more tutorials about software engineering here.
Thanks for reading, please consider tipping some BTC if you liked the blog!
Click or scan the QR code below.
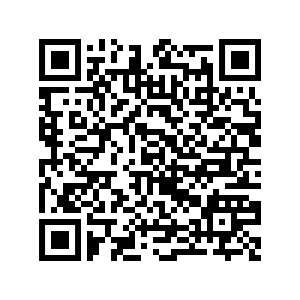