How to Subscribe to The Result of an API Call in Angular
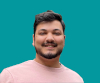
Jacob Naryan - Full-Stack Developer
Posted: Sat Aug 05 2023
Last updated: Wed Nov 22 2023
Angular provides a mechanism to make API calls with its HttpClientModule. When making these calls, you may need to subscribe to the result in a different file than your component. To do this, you can use the Angular services pattern, which allows you to abstract out the logic that makes the API call and subscribe to its result. In this blog post, we’ll discuss how to do this.
1. Create an Angular Service
The first step is to create an Angular service that will be responsible for making the API call and subscribing to its result. Services are typically created in their own .ts files and exported so that they can be imported and used by other components. For this example, let’s create a service called HttpService
:
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class HttpService {
constructor(private http: HttpClient) { }
getData(): Observable<any> {
return this.http.get('http://my-url-here.com/data');
}
}
The HttpService class is decorated with the @Injectable() decorator, which tells Angular that it can be injected into other components as a service. It also has a constructor that takes an instance of HttpClient as an argument, so that we can use it to make our API calls. Finally, it has a getData() method which makes an HTTP GET request and returns an Observable of the response data.
2. Import and Inject the Service into Your Component
Once you have your service created, you can import it into your component and use it. To do this, you need to inject the service into your component’s constructor:
import { Component } from '@angular/core';
import { HttpService } from './http.service'; // import the service here
@Component({ ... })
export class MyComponent {
constructor(private httpService: HttpService) { } // inject the service here
...
}
Now that our service is imported and injected into our component, we can use it to make our API call and subscribe to its result.
3. Subscribe to the Result of the API Call
To subscribe to the result of the API call, you need to call the getData()
method of your HttpService
in your component's ngOnInit()
lifecycle hook and then subscribe to the result:
import { Component, OnInit } from '@angular/core';
import { HttpService } from './http.service'; // import the service here
@Component({ ... })
export class MyComponent implements OnInit {
constructor(private httpService: HttpService) { } // inject the service here
ngOnInit() {
this.httpService.getData().subscribe((data) => {
// do something with the data here
});
}
...
}
Now when our component is initialized, it will make an HTTP GET request and subscribe to its result. This allows us to keep our logic for making API calls and subscribing to its results in a separate file from our component, making our code more maintainable and easier to read.
Thank you for reading.
Thank you for reading. If you liked this blog, check out my personal blog for more content like this.