How to Integrate Your Most Recent Medium Posts into Your Site with JavaScript
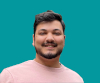
Jacob Naryan - Full-Stack Developer
Posted: Fri Mar 31 2023
Last updated: Wed Nov 22 2023
If you’re a Medium user and you want to access your 10 most recent articles, you can do this using JavaScript / Node.js. This tutorial will explain the steps you need to take in order to get your 10 most recent Medium articles in JavaScript.
1. Install the Required Dependencies
The first step is to install the necessary dependencies for this project. We’ll be using two libraries, axios and cheerio, to make our HTTP request and parse the HTML from the response. To install these libraries, run the following commands in your terminal:
npm install axios cheerio
2. Make an HTTP GET Request
Next, we need to make an HTTP GET request to the URL of our Medium page. We can use the axios library for this:
const axios = require('axios');
const response = await axios.get(`https://medium.com/feed/@jacobnarayan`);
3. Parse the HTML Response
Now that we have the response from our HTTP request, we need to parse the XML so that we can extract the necessary data from it. For that, we’ll use the cheerio library:
const cheerio = require('cheerio');
const $ = cheerio.load(response.data, { xmlMode: true });
4. Get the List of Articles
We can now get the list of articles from our response using the item tag:
const items = $('item')
This will give us a list of our 10 most recent articles on Medium.
5. Extract Data From Each Article
Now that we have all of our articles, we need to extract the data from each article. We can do this by looping through our list of items and extracting the title and content:encoded tags for each item:
var blogs = [] // array to store blog info
items.each((index, item) => { // loop through items
// Extract the <title> and <content> tags for the current <item> tag
const title = $(item).find('title').text(); // extract title
const content = $(item).find('content\\:encoded').text(); // extract content
// create cheerio instance to parse content
var doc = cheerio.load(content);
// add title and content to our blog array
blogs.push({title: title, content: doc.html()})
// Do something with the extracted data, such as log it to the console
});
return blogs; // return array of blog info
And that’s it! Now you have an array containing information about your 10 most recent Medium articles written in JavaScript! To run the program, save this code in a .js file, and in a terminal run node <your-file-name>.js
Additional information such as tags and the post image is also returned, I encourage you to play around with this program to take out the data you need.
Thank you for reading. If you liked this blog check out more tutorials about software engineering here.