How to Integrate Stripe Payments into Your React.js Site
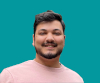
Jacob Naryan - Full-Stack Developer
Posted: Sat Aug 05 2023
Last updated: Wed Nov 22 2023
Are you looking for a way to easily add payment processing to your React.js site? Stripe is one of the most popular payment providers, and it’s easy to integrate into your React.js site using the Stripe.js library and premade components. Here’s a step-by-step guide to get you set up with Stripe payments in no time.
1. Create a File
First, create a file called ‘StripePayment.js’ in the src directory of your React project. This is where you’ll write all the code needed for the Stripe payment integration.
2. Install Stripe and Component Libraries
Next, install the Stripe library and the components library. To do this, open your terminal and run the following commands:
npm install stripe
npm install @stripe/react-stripe-js
3. Write Stripe Code
Next, open up the StripePayment.js file in your text editor and add the following code:
import {Elements} from '@stripe/react-stripe-js';
import {loadStripe} from '@stripe/stripe-js';
// Make sure to call `loadStripe` outside of a component’s render to avoid
// recreating the `Stripe` object on every render.
const stripePromise = loadStripe('pk_test_TYooMQauvdEDq54NiTphI7jx');
The code above imports the Stripe and components libraries, as well as sets up a promise that will be used later on in the code.
4. Create a Form Component
Now it’s time to create a form component that will be used to collect payment information from customers. In your StripePayment.js file, add the following code:
import {PaymentElement} from '@stripe/react-stripe-js';
const CheckoutForm = () => {
return (
<form>
<PaymentElement />
<button>Submit</button>
</form>
);
};
export default CheckoutForm;
The code above creates a form component that will be used to collect payment information from customers. The `handleSubmit()` function is called when the form is submitted and can be used to process the payment information.
5. Render Form Component
Now that you’ve created the form component, you can render it in your React app by adding the following code to your `StripePayment.js` file:
import {Elements} from '@stripe/react-stripe-js';
import {loadStripe} from '@stripe/stripe-js';
// Make sure to call `loadStripe` outside of a component's render to avoid
// recreating the `Stripe` object on every render.
const stripePromise = loadStripe('pk_test_TYooMQauvdEDq54NiTphI7jx');
export default function App() {
const options = {
// passing the client secret obtained from the Stripe Dashboard
clientSecret: '{{CLIENT_SECRET}}',
};
return (
<Elements stripe={stripePromise} options={options}>
<CheckoutForm />
</Elements>
);
};
6. Test Payment Integration
Now that you’ve integrated Stripe into your React app, you can test it out by running it locally and submitting a payment form with test credit card details (available in your Stripe dashboard). If everything works correctly then you should see a success message when submitting the form! Congratulations — you’ve just integrated Stripe payments into your React app!
Thank you for reading. If you liked this blog, check out my personal blog for more content about software engineering and technology topics!