How to Easily Create a Popup With Angular Material
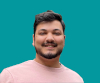
Jacob Naryan - Full-Stack Developer
Posted: Sat Jul 22 2023
Last updated: Wed Nov 22 2023
Are you looking to create a popup in your Angular app using Typescript? Angular Material is the perfect tool for creating popups in your app and in this blog, I will show you how.
1. Install the Necessary Dependencies
The first step is to install @angular/material.
ng add @angular/material
or
npm install — save @angular/material @angular/cdk
2. Import the Necessary Modules
Once you have installed the dependencies, you will need to import them into your app. You can do this by adding the following code to your app.module.ts file:
import {MatDialogModule} from '@angular/material/dialog';
import {MatButtonModule} from '@angular/material/button';
Then, add these modules to your imports array:
imports: [
...
MatDialogModule,
MatButtonModule,
...
]
3. Create a Dialog Component
The next step is to create a dialog component. This component will be responsible for creating and displaying the dialog. To do this, create a new component with the following command:
ng generate component dialog-component
4. Create a Template for the Dialog Component
Next, you will need to create an HTML template for the dialog component. This template will be responsible for displaying the content of your dialog. Here’s an example of what this template might look like:
<h1 mat-dialog-title>Hello {{ data.name }}</h1>
<mat-dialog-content>This is my dialog content!</mat-dialog-content>
<mat-dialog-actions>
<button mat-button mat-dialog-close>Close</button>
</mat-dialog-actions>
5. Create a Dialog Service
The next step is to create a service that will be responsible for opening the dialog. This service will also be responsible for passing any data that needs to be displayed in the dialog. Here’s an example of what this service might look like:
import { Injectable } from '@angular/core';
import { MatDialog } from '@angular/material/dialog';
//component we just created
import {PopupComponent} from '../app/components/common/popup/popup.component'
@Injectable()
export class DialogService {
constructor(private dialog: MatDialog) {}
open(data: any) {
return this.dialog.open(PopupComponent, {
data: data
});
}
}
6. Use Your Dialog Service
The last step is to inject your service into a component and use it. In your constructor add the service you just created like this.
constructor(private _dialog: DialogService){
}
Then use the service like this:
//open the dialog
var ref = this._dialog.open({name: "First Last"});
//close the dialog
ref.close()
Conclusion
Creating a dialog with Angular Material and Typescript is a simple and straightforward process. We hope this tutorial has been helpful in showing you how to get started with creating your own dialogs in your Angular apps!
Thank you for reading. If you liked this blog check out more tutorials about software engineering here.
Thanks for reading, please consider tipping some BTC if you liked the blog!
Click or scan the QR code below.
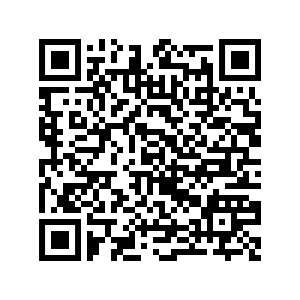