How to Create a Scroll Animation in React.js with Framer Motion Library
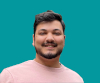
Jacob Naryan - Full-Stack Developer
Posted: Thu Aug 31 2023
Last updated: Wed Nov 22 2023
In this tutorial, we will walk you through the process of creating a scroll animation in React.js, using the Framer Motion library. This code will let you run an animation on a React component when it comes into the viewport.
1. Prerequisites
Before we get started, ensure that you have Node.js and npm installed on your computer. You also need to have a basic understanding of React.js and JavaScript.
2. Installing Framer Motion
The next step is installing the Framer Motion library. Run this command to install:
npm install framer-motion
3. Writing the Scroll Animation Code
In ScrollAnimation.js
, start by importing React and framer-motion and creating a functional component:
import React from 'react';
import { motion } from "framer-motion";
const ScrollAnimation = () => {
return (
<motion.div
initial={{ opacity: 0, y: 50 }}
whileInView={{ opacity: 1, y:0 }}
viewport={{once:true}}
transition={{
ease: "linear",
duration: 1,
y: { duration: 0.5 }
}}
>
<h1>Scroll Down!</h1>
</motion.div>
)
}
export default ScrollAnimation;
This code sets the initial opacity of the element to 0 and offsets the y-axis by 50px. While it’s in view, it will set the opacity to 1 and set the y-axis to 0 which will give it the effect of fading in from the bottom. We’ve also set this animation to run once when it first comes into the viewport, to ease into the animation, and to take a total time of 0.5 seconds.
6. Implementing the Scroll Animation Component
In your App.js
file, import your ScrollAnimation
component and add it to your render method:
import React from 'react';
import './App.css';
import ScrollAnimation from './ScrollAnimation';
function App() {
return (
<div className="App">
<ScrollAnimation />
</div>
);
}
export default App;
7. Testing Your Scroll Animation
Finally, run your app with:
npm start
Navigate to http://localhost:3000
in your browser to see your newly created scroll animation in action!
This is just a simple example, but you can customize your animations any way you like using Framer Motion’s API.
Thank you for reading. If you liked this blog, check out more of my tutorials about software engineering on my personal site here.