How to Make a Custom Form Validator with Angular Reactive Forms
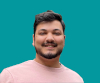
Jacob Naryan - Full-Stack Developer
Posted: Tue Jul 25 2023
Last updated: Wed Nov 22 2023
Angular Reactive Forms provide a powerful way to create and manage forms in your application. By combining the power of Angular forms with custom, tailored validators, you can create a robust and user-friendly experience for your users. In this tutorial, we’ll go over how to make a custom form validator with Angular Reactive Forms.
1. Create a FormGroup
The first step in creating a custom form validator is to create the form group that will contain all of the form controls. We do this using the
FormBuilderclass and the
group()method. This method takes an object as an argument, with each key representing a form control.
// import FormBuilder from '@angular/forms'
constructor(private fb: FormBuilder) {}
// create the form group
this.form = this.fb.group({
myField: new FormControl('', [Validators.Required, MyCustomValidator])
})
Each form control requires two arguments: the initial value and an array of validators for that control. We can also include our custom validator in the array of validators. Here we are using the built-in validator ‘Validators.Required’, and a custom validator that we will create ‘MyCustomValidator’
2. Create the Custom Validator Function
Next, we’ll create the custom validator function that will be used to validate our form control. This is a simple function that takes in the value of the control and returns either null or an object with an error message depending on whether or not it is valid.
// create the custom validator function
function MyCustomValidator(control: AbstractControl){
// check if value is valid or not
const >// ...some logic here
// returns null if value is valid, or an error message otherwise
return isValid ? null : { 'myCustomError': 'This value is invalid' };
}
The custom validator function should return null if the value is valid, or an object containing an error message if it is not valid. We can then use this function in our form control to validate the value entered by the user.
3. Add an Error Message to Your Form
Once we have created our custom validator function, any value entered by the user will be validated against our custom validation logic before being accepted by the form control. This will allow you to provide real-time feedback to users if what they entered is valid. This will help us create a more robust and user-friendly experience for our users.
<form [formGroup]='form'>
<label>Field</label>
<input type='text' formControlName='myField' >
<div *ngIf='form.get('myField').touched) && form.get('myField').invalid'>
There is a problem with your input
</div>
<button>Submit</button>
</form>
4. Test Your Validator
Finally, it’s always a good idea to test your custom validators before deploying them in production. We can do this by creating a simple test case and running it through our custom validation logic to make sure it works as expected. This will help us catch any potential issues before they become problems in production and ensure that our custom validation logic is working as expected.
With these steps completed, we have now successfully created a custom form validator with Angular Reactive Forms! By combining the power of Angular forms with custom, tailored validators, we can create a robust and user-friendly experience for our users and ensure that any data entered into our forms is validated correctly and securely.