How to Combine an Audio and Video File With 7 Lines of Python
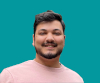
Jacob Naryan - Full-Stack Developer
Posted: Sat Apr 01 2023
Last updated: Wed Nov 22 2023
Have you ever needed to add a voice-over or some other audio track over a video? Well, there is a pretty simple way to automate this video editing task with a few lines of Python code.
1. Set Up
First, we need to import the required modules from moviepy
. We'll be using VideoFileClip
, AudioFileClip
, and concatenate_videoclips
. We'll also need to import the os
module to access the directory of our files.
from moviepy.editor import VideoFileClip, AudioFileClip, concatenate_videoclips
import os
2. Get the Title
Next, we’ll ask the user for a title for the new video. This will be used to name the final video file.
# Get the desired video title
title = input("Enter a title: ")
3. Open the Video and Audio Files
Now we’ll open the video and audio files that we want to combine. We’ll use VideoFileClip
and AudioFileClip
to open the files.
# Open the video and audio
video_clip = VideoFileClip("video.mp4")
audio_clip = AudioFileClip("audio.mp3")
4. Concatenate Clips
Once we have opened the clips, we can combine them using concatenate_videoclips
. This will combine the video clip with the audio clip to create a single clip with both elements.
# Concatenate the video clip with the audio clip
final_clip = video_clip.set_audio(audio_clip)
5. Export Video
Finally, we can use write_videofile
to export the finished video with audio as an .mp4 file. The title that was entered earlier will be used as the file name for easy identification.
# Export the final video with audio
final_clip.write_videofile(title + ".mp4")
And that’s it! You’ve successfully combined your audio and video.
Watch the video tutorial here:
Thank you for reading. If you liked this blog check out more tutorials about software engineering here.
Thanks for reading, please consider tipping some BTC if you liked the blog!
Click or scan the QR code below.
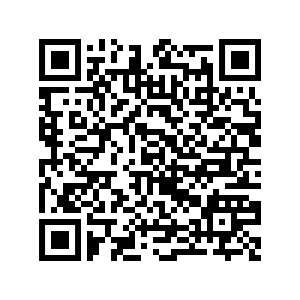