How to Buy and Sell Stocks With Python
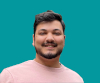
Jacob Naryan - Full-Stack Developer
Posted: Sat Aug 05 2023
Last updated: Wed Nov 22 2023
Alpaca Markets Trading API and Python can be used to easily buy and sell stocks. In this blog post, I will walk you through the steps needed to buy and sell a stock using the Alpaca Markets Trading API and Python.
1. Setting Up Alpaca Markets Trading API
The first step is to set up the Alpaca Markets Trading API. You will need to create an Alpaca account and provide your API key ID, API secret key, paper key, and paper secret. You will also need to set up both the test and live endpoints for the Alpaca Markets Trading API. I’d recommend putting your credentials in a config file and importing like so:
import alpaca_trade_api as tradeapi
import time
from config import APCA_API_KEY_ID, APCA_API_SECRET_KEY, APCA_PAPER_KEY, APCA_PAPER_SECRET, alpaca_test_endpoint, alpaca_endpoint
trader = tradeapi.REST(key_id=APCA_API_KEY_ID, secret_key=APCA_API_SECRET_KEY, base_url=alpaca_endpoint)
2. Submitting a Buy Order
Once the Alpaca Markets Trading API is set up, you can use Python to submit a buy order. You will need to specify the symbol (e.g ETHUSD), side (buy), quantity, type (market), and time in force (GTC). After submitting the buy order, you can use Python to retrieve the order’s ID and status.
quantity_bought = 0
position_price = 0
amount_paid = 0
buy = trader.submit_order(
symbol='TSLA',
side='buy',
qty=1,
type='market',
time_in_force='gtc'
)
buy_id = buy.id
buy_order_status = buy.status
Here we are submitting a market buy order for 1 Tesla stock, the order is good till canceled. We are then getting the order ID and current status.
3. Wait Until The Order is Fulfilled
Once you’ve placed your order, check every second until the order is fulfilled. Once it is, set variables for how much you bought, at what price, and the total amount paid.
while True:
time.sleep(1)
order = trader.get_order(buy_id)
buy_order_status = order.status
if order.status == 'filled':
quantity_bought = float(order.filled_qty)
position_price = float(order.filled_avg_price)
amount_paid = position_price * quantity_bought
break
4. Implementing Your Trading Strategy
Once you have successfully submitted a buy order, you can implement your trading strategy. This will depend on your individual strategy but could include analyzing price movements or tracking news events related to the stock.
5. Submitting a Sell Order
After implementing your trading strategy, you can then submit a sell order using Python. This will be similar to submitting a buy order, but with a few changes — you will specify the side as “sell” and the quantity as the quantity bought in step 2. Once you have submitted the sell order, you can retrieve its status as we did in step 3.
sell = trader.submit_order(
symbol='TSLA',
side='sell',
qty=quantity_bought,
type='market',
time_in_force='gtc'
)
Conclusion
Buying and selling stocks with Alpaca Markets Trading API and Python is easy and straightforward. By following these steps, you can quickly get started on your stock trading journey!
Watch the video tutorial here:
Thank you for reading. If you liked this blog, check out my personal blog for more content about software engineering and technology topics!