How to Automate Your Authentication Flow in Postman
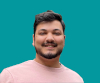
Jacob Naryan - Full-Stack Developer
Posted: Sat Aug 05 2023
Last updated: Wed Nov 22 2023
Postman is a great tool for developers that allows them to test their APIs. It also provides features to automate authentication flows with the pre-request tab, collection or global variables, and the pm library. With these advanced features, you can easily set up and maintain authentication for your API requests. Here’s a step-by-step guide on how to do it.
1. Set Collection and Global Variables
You can use Postman’s collection and global variables to store and manage the authentication information. To do this, go to the “Manage Environments” tab in Postman and click on “Add” to create a new environment. Then, add the authentication information as variables in this environment. You can use these variables in any request that you make in Postman.
2. Use the Pre-Request Script Tab
Next, open the “Pre-request Script” tab in Postman and add code to authenticate your API requests. You can use the collection or global variables that you set earlier to store the authentication information, and then use the built-in pm library to send requests and set/retrieve the token expiration timestamp and auth token. This code will be run before every request, so you don’t have to worry about manually authenticating each time.
var accessToken = pm.globals.get("accessToken");
var tokenExpiry = pm.globals.get("tokenExpiry");
var username = pm.environment.get("username");
var password = pm.environment.get("password");
// Check if the access token is expired or null
if (!accessToken || new Date() > new Date(tokenExpiry)) {
// Send a POST request to the authentication endpoint
pm.sendRequest({
url: "https://your-auth-endpoint.com/login",
method: "POST",
header: {
"Content-Type": "application/json"
},
body: {
mode: "raw",
raw: JSON.stringify({
username: username,
password: password
})
}
}, function (err, res) {
// Check if the request was successful
if (err === null && res.code === 200) {
// Parse the response body
var responseJson = JSON.parse(res.body);
// Set the token and expiration date as collection variables
pm.globals.set("accessToken", responseJson.accessToken);
pm.globals.set("tokenExpiry", responseJson.expiresAt);
console.log("Access token and expiry set as collection variables");
} else {
console.error("Request failed: " + err);
}
});
} else {
console.log("Access token is still valid, no request sent");
}
This code grabs the access token, token expiration timestamp, username, and password from the global and environment variables. It then checks if there is no token or if the token is expired. If either is true it will fetch a new token and update the global variables.
Note: Replace ‘https://your-auth-endpoint.com/login’ with the actual URL of your authentication endpoint.
3. Set Your Authentication Headers
Once you have this code in your pre-request tab, set your authorization token in the authorization tab by including {{accessToken}} as the value of your authorization token.
4. Test Your Requests
Finally, you can test your API requests with Postman to make sure everything is working correctly. You should also make sure that your authentication is working correctly by checking the response codes of your requests in the console or the response tab. If everything looks good, then you’ve successfully automated your authentication flow!
Thank you for reading. If you liked this blog, check out my personal blog for more content about software engineering and technology topics!