How to Add a Script to Specific Pages in Next.js Using the Script Tag and ‘Only’ Attribute
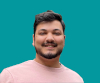
Jacob Naryan - Full-Stack Developer
Posted: Sat Aug 12 2023
Last updated: Wed Nov 22 2023
One powerful feature of Next.js is the ability to attach scripts to specific pages using the Script tag and the ‘only’ attribute. This blog post will walk you through the steps on how to do this. I’m writing this because I recently was trying to load a script to a specific page on my Next.js site and found this information difficult to find.
1. Understanding the Next.js Script Tag
The Next.js Script tag is a built-in component that helps with loading either external or inline scripts in your Next.js application. It provides several strategies to optimize loading for third-party scripts which can significantly improve your site’s performance.
To add a script, you will typically use the Script tag as follows:
import Script from 'next/script'
function MyComponent() {
return (
<div>
<Script src="https://www.example.com/script.js" />
</div>
)
}
In this snippet, src
is the source URL of the external script you want to load.
2. Introducing the ‘only’ Attribute
While it’s often helpful to load scripts globally, there might be cases where you want to load a script only on specific pages. This is where the ‘only’ attribute comes in handy.
The ‘only’ attribute allows you to specify that a script should only be loaded on a certain page or set of pages. This can optimize your site’s performance by reducing unnecessary network requests and JavaScript execution on pages where a script isn’t needed.
Here’s an example of how you might use it:
import Script from 'next/script'
function MyComponent() {
return (
<div>
<Script only="examplePage" src="https://www.example.com/script.js" />
</div>
)
}
In this example, the script will only be loaded when visiting examplePage
.
3. Adding a Script to Specific Pages
Now that we understand how the Next.js Script tag works and what the ‘only’ attribute does, let’s look at how we can use them together to add a script to specific pages.
Let’s say we have a script that we only want to load on our Home and About pages. Here’s how we could do it:
import Script from 'next/script'
function HomeComponent() {
return (
<div>
<Script only="home" src="https://www.example.com/home-script.js" />
</div>
)
}
function AboutComponent() {
return (
<div>
<Script only="about" src="https://www.example.com/about-script.js" />
</div>
)
}
In these examples, home-script.js
will only load when visiting the Home page, and about-script.js
will only load when visiting the About page.
This is a powerful feature that can greatly improve your site’s performance by ensuring scripts are only loaded when they’re needed.
Thank you for reading. If you liked this blog, check out more of my tutorials about software engineering on my personal site here.